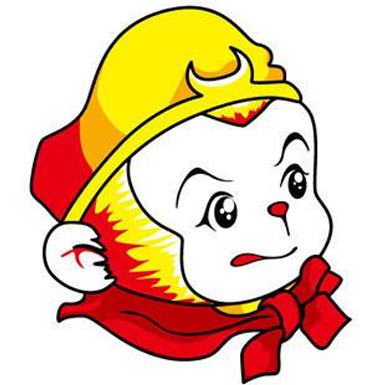
To prevent using macros before they are defined, enfoce calling them in an appropriate order. Signed-off-by: Michal Mielewczyk <michal.mielewczyk@intel.com>
86 lines
2.6 KiB
Bash
86 lines
2.6 KiB
Bash
#!/bin/bash
|
|
#
|
|
# Copyright(c) 2012-2019 Intel Corporation
|
|
# SPDX-License-Identifier: BSD-3-Clause-Clear
|
|
#
|
|
|
|
. `dirname $0`/conf_framework
|
|
|
|
if
|
|
compile_module "generic_start_io_acct(NULL, 0, 0, NULL)" "linux/bio.h"
|
|
then
|
|
add_function "
|
|
static inline void cas_generic_start_io_acct(struct request_queue *q,
|
|
int rw, unsigned long sectors, struct hd_struct *part) {
|
|
generic_start_io_acct(q, rw, sectors, part);
|
|
}"
|
|
add_function "
|
|
static inline void cas_generic_end_io_acct(struct request_queue *q,
|
|
int rw, struct hd_struct *part, unsigned long start_time)
|
|
{
|
|
generic_end_io_acct(q, rw, part, start_time);
|
|
}"
|
|
elif compile_module "generic_start_io_acct(0, 0, NULL)" "linux/bio.h"
|
|
then
|
|
add_function "
|
|
static inline void cas_generic_start_io_acct(struct request_queue *q,
|
|
int rw, unsigned long sectors, struct hd_struct *part)
|
|
{
|
|
generic_start_io_acct(rw, sectors, part);
|
|
}"
|
|
add_function "
|
|
static inline void cas_generic_end_io_acct(struct request_queue *q,
|
|
int rw, struct hd_struct *part, unsigned long start_time)
|
|
{
|
|
generic_end_io_acct(rw, part, start_time);
|
|
}"
|
|
elif compile_module "part_round_stats(1, 1)" "linux/genhd.h"
|
|
then
|
|
add_function "
|
|
static inline void cas_generic_start_io_acct(struct request_queue *q,
|
|
int rw, unsigned long sectors, struct hd_struct *part)
|
|
{
|
|
int cpu = part_stat_lock();
|
|
part_round_stats(cpu, part);
|
|
part_stat_inc(cpu, part, ios[rw]);
|
|
part_stat_add(cpu, part, sectors[rw], sectors);
|
|
part_inc_in_flight(part, rw);
|
|
part_stat_unlock();
|
|
}"
|
|
add_function "
|
|
static inline void cas_generic_end_io_acct(struct request_queue *q,
|
|
int rw, struct hd_struct *part, unsigned long start_time)
|
|
{
|
|
unsigned long duration = jiffies - start_time;
|
|
int cpu = part_stat_lock();
|
|
part_stat_add(cpu, part, ticks[rw], duration);
|
|
part_round_stats(cpu, part);
|
|
part_dec_in_flight(part, rw);
|
|
part_stat_unlock();
|
|
}"
|
|
elif compile_module "part_round_stats(NULL, 1, 1)" "linux/genhd.h"
|
|
then
|
|
add_function "
|
|
static inline void cas_generic_start_io_acct(struct request_queue *q,
|
|
int rw, unsigned long sectors, struct hd_struct *part)
|
|
{
|
|
int cpu = part_stat_lock();
|
|
part_round_stats(NULL, cpu, part);
|
|
part_stat_inc(cpu, part, ios[rw]);
|
|
part_stat_add(cpu, part, sectors[rw], sectors);
|
|
part_inc_in_flight(NULL, part, rw);
|
|
part_stat_unlock();
|
|
}"
|
|
add_function "
|
|
static inline void cas_generic_end_io_acct(struct request_queue *q,
|
|
int rw, struct hd_struct *part, unsigned long start_time)
|
|
{
|
|
unsigned long duration = jiffies - start_time;
|
|
int cpu = part_stat_lock();
|
|
part_stat_add(cpu, part, ticks[rw], duration);
|
|
part_round_stats(NULL, cpu, part);
|
|
part_dec_in_flight(NULL, part, rw);
|
|
part_stat_unlock();
|
|
}"
|
|
fi
|